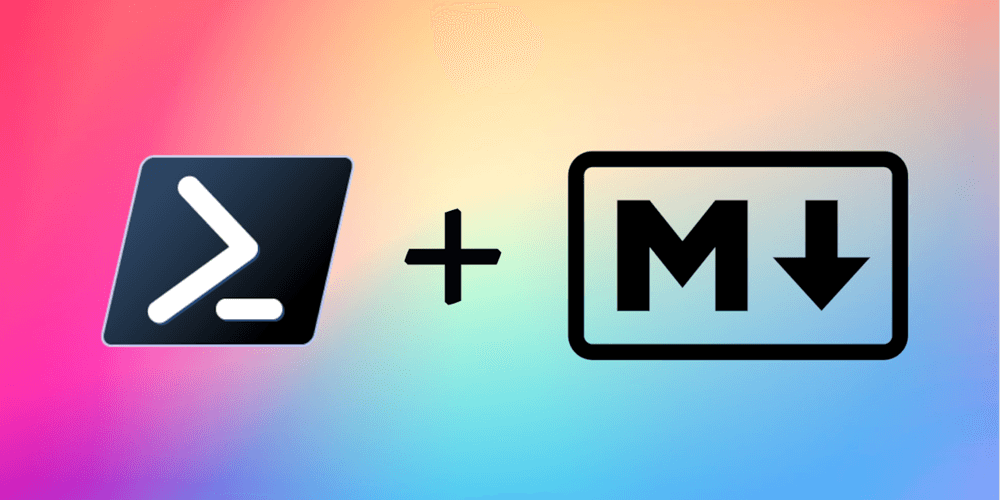
PSMarkdownTable
Photo Credits: Unsplash and Tech Icons
Introduction
My most controversial preference as a command-line user is probably the one I have for PowerShell over Bash. When an explanation is demanded, I typically retort with the (hyperbolic) line:
In Bash, everything's a string. In PowerShell, everything's an object.
While neither statement is fully true, I consider them representative of each language's approach.
I find that the object-oriented nature of PowerShell makes common tasks much easier, or - at the very least - much more intuitive. Not only can you build and work with complex data structures, but PowerShell offers built-in cmdlets for serialization and de-serialization, including my favorites ConvertFrom-Json
, ConvertTo-Json
, ConvertFrom-Csv
, and ConvertTo-Csv
.
This project creates a similar module for serializing to and de-serializing from Markdown tables.
It's certainly a more niche use case, and it only works for simple data structures; However, I often find myself taking notes that I'd like to be able to later read or work with programmatically. I want my data to be both structured (for easy parsing, searching, and manipulation) and pleasantly readable (i.e. not JSON, yaml, csv, etc.). The solution I've settled on - this module - allows me to record data in any de-serializable format (now including Markdown tables), then convert it to any other (such as JSON).
Examples
JSON --> PowerShell --> Markdown
Given a JSON file like the following:
[
{
"prop2": "a property",
"prop1": 1,
"prop3": "hello"
},
{
"prop2": 5,
"prop1": "world",
"prop3": "another"
}
]
We can de-serialize the JSON using PowerShell's ConvertFrom-JSON
:
PS:> Get-Content .\test.json | ConvertFrom-Json | Format-Table -Property prop1, prop2, prop3
prop1 prop2 prop3
----- ----- -----
1 a property hello
world 5 another
Once the data is de-serialized into a PowerShell array, we can re-serialize it using my module:
PS:> Import-Module .\PSMarkdownTable\PSMarkdownTable.psm1
PS:> Get-Content .\test.json | ConvertFrom-Json | ConvertTo-MdTable
| prop2 | prop1 | prop3 |
| ---------- | ----- | ------- |
| a property | 1 | hello |
| 5 | world | another |
Note: At the time of writing, I have not incorporated a way to specify the column order of object properties
Markdown --> PowerShell --> CSV
Given the same data as before, we can de-serialize a Markdown table, then re-serialize into (for example) the CSV format.
Note: If jumping over the previous section, remember to import the module before running these commands.
PS:> $md = @"
| prop2 | prop1 | prop3 |
| ---------- | ----- | ------- |
| a property | 1 | hello |
| 5 | world | another |
"@
PS:> $md | ConvertFrom-MdTable
prop2 prop1 prop3
----- ----- -----
a property 1 hello
5 world another
PS:> $md | ConvertFrom-MdTable | ConvertTo-Csv
#TYPE System.Management.Automation.PSCustomObject
"prop2","prop1","prop3"
"a property","1","hello"
"5","world","another"
Source Code
You can find the source code for the PowerShell module here.